Isochrone Object¶
The isochrone classes are defined in spisea/synthetic.py
. The primary
inputs to a isochrone object are the stellar population age, distance,
total extinction, and metallicity, along with the Atmosphere Model Object,
Evolution Model Object, and Extinction Law Object.
If the IsochronePhot sub-class is used then synthetic photometry will be produced. The Photometric Filters are defined as additional inputs.
An example of making an IsochronePhot object:
from spisea import synthetic, evolution
from spisea import atmospheres, reddening
import numpy as np
# Define isochrone input parameters
logAge = np.log10(5*10**6.) # Age in log(years)
dist = 4000 # distance in parsec
AKs = 0.8 # extinction in Ks-band mags
metallicity = 0 # Metallicity in [M/H]
evo_model = evolution.MISTv1()
atm_func = atmospheres.get_merged_atmosphere
red_law = reddening.RedLawHosek18b()
# Specify filters for synthetic photometry. Here we
# use the HST WFC3-IR F127M, F139M, and F153M filters
filt_list = ['wfc3,ir,f127m', 'wfc3,ir,f139m',
'wfc3,ir,f153m']
# Specify the directory we want the output isochrone
# table saved in
iso_dir = './isochrones/'
# Make IsochronePhot object
my_iso = synthetic.IsochronePhot(logAge, AKs, dist,
metallicity=0,
evo_model=evo_model,
atm_func=atm_func,
red_law=red_law,
filters=filt_list,
iso_dir=iso_dir)
See Quick Start Example for a detailed example showing how to interact with the isochrone object output. Here is a table from Hosek et al. 2020 that describes the values in the isochrone output table:
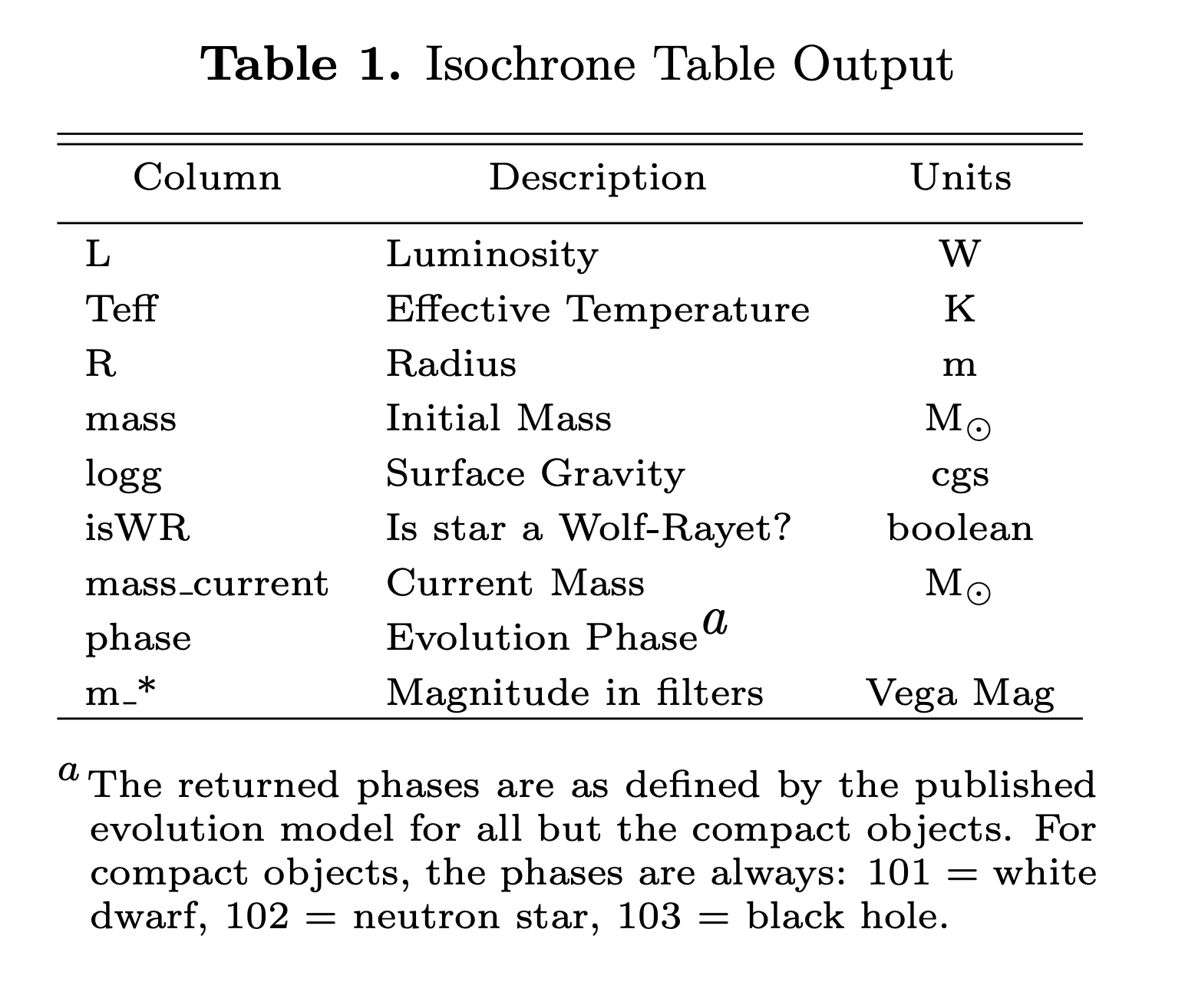
Tips and Tricks: The IsochronePhot Object¶
It takes ~1-3 mins to make an IsochronePhot object for the first time. A FITS table is created with the stellar parameters and photometry for each star. This table is saved in the specified iso_dir, under the filename iso_<age>_<aks>_<dist>_<z>.fits. By default, iso_dir is set to the current working directory unless otherwise defined.
In future calls of IsochronePhot, the code will first check iso_dir to see if the appropriate FITS table already exists. If so, then it will simply read the table and be done. This saves significant amounts of computation time.
WARNING: When IsochronePhot checks to see if the desired isochrone table already exists, it checks all isochrone properties except for the photometric filters (evolution models, atmosphere models, and reddening law are encoded in the table meta-data). If any of these parameters do not match, then the isochrone will be re-calculated.
However, to keep the isochrone filenames reasonable, only the age, extinction, distance, and metallicity are encoded in the filename itself. So, if the evolution model, atmosphere model, or reddening law have changed, the original file will be overwritten by the new isochrone.
To avoid files from being unintentially overwritten, we recommend that users specify different iso_dir paths when making isochrones with different evolution models, atmosphere models, or reddening laws.
WARNING: IsochronePhot does not check existing isochrone tables to see if the photometric filters match those specified by the user. So, if the user wishes to generate an isochrone with different filters, we recommend either using a different iso_dir path or setting the keyword recomp=True (see docs below).
Base Isochrone Class¶
- class synthetic.Isochrone(logAge, AKs, distance, metallicity=0.0, evo_model=<spisea.evolution.MISTv1 object>, atm_func=<function get_merged_atmosphere>, wd_atm_func=<function get_wd_atmosphere>, red_law=<spisea.reddening.RedLawNishiyama09 object>, mass_sampling=1, wave_range=[3000, 52000], min_mass=None, max_mass=None, rebin=True)¶
Base Isochrone class.
- Parameters
- logAgefloat
The age of the isochrone, in log(years)
- AKsfloat
The total extinction in Ks filter, in magnitudes
- distancefloat
The distance of the isochrone, in pc
- metallicityfloat, optional
The metallicity of the isochrone, in [M/H]. Default is 0.
- evo_model: model evolution class, optional
Set the stellar evolution model class. Default is evolution.MISTv1().
- atm_func: model atmosphere function, optional
Set the stellar atmosphere models for the stars. Default is get_merged_atmosphere.
- wd_atm_func: white dwarf model atmosphere function, optional
Set the stellar atmosphere models for the white dwafs. Default is get_wd_atmosphere
- mass_samplingint, optional
Sample the raw isochrone every mass_sampling steps. The default is mass_sampling = 0, which is the native isochrone mass sampling of the evolution model.
- wave_rangelist, optional
length=2 list with the wavelength min/max of the final spectra. Units are Angstroms. Default is [3000, 52000].
- min_massfloat or None, optional
If float, defines the minimum mass in the isochrone. Unit is solar masses. Default is None
- max_massfloat or None, optional
If float, defines the maxmimum mass in the isochrone. Units is solar masses. Default is None.
- rebinboolean, optional
If true, rebins the atmospheres so that they are the same resolution as the Castelli+04 atmospheres. Default is False, which is often sufficient synthetic photometry in most cases.
Methods
plot_HR_diagram
([savefile])Make a standard HR diagram for this isochrone.
plot_mass_luminosity
([savefile])Make a standard mass-luminosity relation plot for this isochrone.
- plot_HR_diagram(savefile=None)¶
Make a standard HR diagram for this isochrone.
- Parameters
- savefile: path or None, optional
Path to file plot too, if desired. Default is None
- plot_mass_luminosity(savefile=None)¶
Make a standard mass-luminosity relation plot for this isochrone.
- Parameters
- savefile: path or None, optional
Path to file plot too, if desired. Default is None
Isochrone Sub-classes¶
- class synthetic.IsochronePhot(logAge, AKs, distance, metallicity=0.0, evo_model=<spisea.evolution.MISTv1 object>, atm_func=<function get_merged_atmosphere>, wd_atm_func=<function get_wd_atmosphere>, wave_range=[3000, 52000], red_law=<spisea.reddening.RedLawNishiyama09 object>, mass_sampling=1, iso_dir='./', min_mass=None, max_mass=None, rebin=True, recomp=False, filters=['ubv,U', 'ubv,B', 'ubv,V', 'ubv,R', 'ubv,I'])¶
Bases:
Isochrone
Make an isochrone with synthetic photometry in various filters. Load from file if possible.
- Parameters
- logAgefloat
The age of the isochrone, in log(years)
- AKsfloat
The total extinction in Ks filter, in magnitudes
- distancefloat
The distance of the isochrone, in pc
- metallicityfloat, optional
The metallicity of the isochrone, in [M/H]. Default is 0.
- evo_model: model evolution class, optional
Set the stellar evolution model class. Default is evolution.MISTv1().
- atm_func: model atmosphere function, optional
Set the stellar atmosphere models for the stars. Default is atmospheres.get_merged_atmosphere.
- wd_atm_func: white dwarf model atmosphere function, optional
Set the stellar atmosphere models for the white dwafs. Default is atmospheres.get_wd_atmosphere
- red_lawreddening law object, optional
Define the reddening law for the synthetic photometry. Default is reddening.RedLawNishiyama09().
- iso_dirpath, optional
Path to isochrone directory. Code will check isochrone directory to see if isochrone file already exists; if it does, it will just read the isochrone. If the isochrone file doesn’t exist, then save isochrone to the isochrone directory.
- mass_samplingint, optional
Sample the raw isochrone every mass_sampling steps. The default is mass_sampling = 0, which is the native isochrone mass sampling of the evolution model.
- wave_rangelist, optional
length=2 list with the wavelength min/max of the final spectra. Units are Angstroms. Default is [3000, 52000].
- min_massfloat or None, optional
If float, defines the minimum mass in the isochrone. Unit is solar masses. Default is None
- max_massfloat or None, optional
If float, defines the maxmimum mass in the isochrone. Units is solar masses. Default is None.
- rebinboolean, optional
If true, rebins the atmospheres so that they are the same resolution as the Castelli+04 atmospheres. Default is True, which is often sufficient synthetic photometry in most cases.
- recompboolean, optional
If true, recalculate the isochrone photometry even if the savefile exists. You should recompute anytime you change the filter set (see filters below).
- filtersarray of strings, optional
Define what filters the synthetic photometry will be calculated for, via the filter string identifier.
Methods
check_save_file
(evo_model, atm_func, red_law)Check to see if save_file exists, as saved by the save_file and save_file_legacy objects.
make_photometry
([rebin, vega])Make synthetic photometry for the specified filters.
plot_CMD
(mag1, mag2[, savefile])Make a CMD with mag1 vs mag1 - mag2
plot_HR_diagram
([savefile])Make a standard HR diagram for this isochrone.
plot_mass_luminosity
([savefile])Make a standard mass-luminosity relation plot for this isochrone.
plot_mass_magnitude
(mag[, savefile])Make a standard mass-luminosity relation plot for this isochrone.