Evolution Model Object¶
Stellar evolution models are defined as classes in
spisea/evolution.py
. These can be called by:
from spisea import evolution
evo = evolution.<model_name>()
The evolution object is an input for the Isochrone Object.
Below is a table of the evolution model grids currently supported by SPISEA.
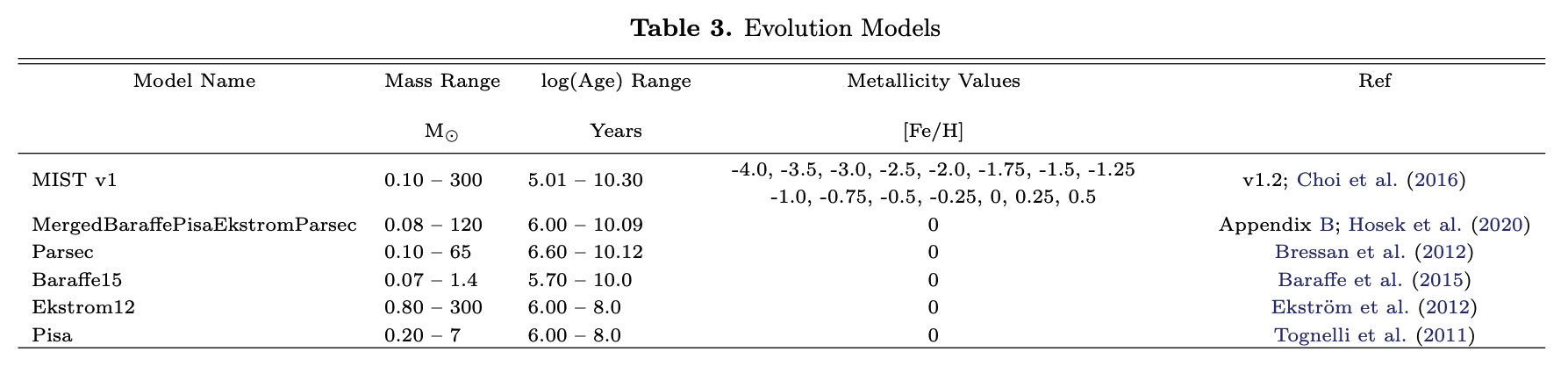
Please note the stellar mass range, age range, and metallicity values of the evolution model grid you choose:
Stars generated from the IMF Object (controlled by the massLimits variable) that fall outside of the mass range of the evolution model will be dropped from the final cluster table, unless the IFMR is defined (which will catch all the high mass stars and get their compact object values)
SPISEA will throw an error if you request an age outside of the evolution model age range
SPISEA will use the evolution model with the closest available metallicity to the requested value
If you require other evolution models or need to expand the existing grids, please see Adding Evolution Models.
ModelMismatch Error¶
Since SPISEA v2.1.4, we began tracking the version of the evolution
model grid (e.g., the evolution models stored in
<SPISEA_MODELS>/evolution
).
Each evolution model class has a required model grid
version assigned to it. If your evolution model grid
does not match or exceed the minimum version required by
your desired evolution model, a ModelMismatch
exception will be raised.
To resolve the ModelMismatch
error, please
re-download the latest version of the evolution
model grid in the installation instructions (Stellar Evolution and Atmosphere Models).
Base Evolution Model Class¶
- class evolution.StellarEvolution(model_dir, age_list, mass_list, z_list)¶
Base Stellar evolution class.
- Parameters
- model_dir: path
Directory path to evolution model files
- age_list: list
List of ages
- mass_list: list
List of masses
- z_list: list
List of metallicities
Specific Evolution Model Classes¶
- class evolution.MISTv1(version=1.2)¶
Bases:
StellarEvolution
Define intrinsic properties for the MIST v1 stellar models.
Models originally downloaded from online server.
- Parameters
- version: ‘1.0’ or ‘1.2’, optional
Specify which version of MIST models you want. Version 1.0 was downloaded from MIST website on 2/2017, while Version 1.2 was downloaded on 8/2018 (solar metallicity) and 4/2019 (other metallicities). Default is 1.2.
Methods
format_isochrones
()Parse isochrone file downloaded from MIST web server, create individual isochrone files for the different ages.
isochrone
([age, metallicity])Extract an individual isochrone from the MISTv1 collection.
- class evolution.MergedBaraffePisaEkstromParsec(rot=True)¶
Bases:
StellarEvolution
This is a combination of several different evolution models:
Baraffe (Baraffe et al. 2015)
Pisa (Tognelli et al. 2011)
Geneva (Ekstrom et al. 2012)
Parsec (version 1.2s, Bressan+12)
The model used depends on the age of the population and what stellar masses are being modeled:
For logAge < 7.4:
Baraffe: 0.08 - 0.4 M_sun
Baraffe/Pisa transition: 0.4 - 0.5 M_sun
Pisa: 0.5 M_sun to the highest mass in Pisa isochrone (typically 5 - 7 Msun)
Geneva: Highest mass of Pisa models to 120 M_sun
For logAge > 7.4:
Parsec v1.2s: full mass range
- Parameters
- rot: boolean, optional
If true, then use rotating Ekstrom models. Default is true.
Methods
isochrone
([age, metallicity])Extract an individual isochrone from the Baraffe-Pisa-Ekstrom-Parsec collection
- class evolution.Baraffe15¶
Bases:
StellarEvolution
Evolution models published in Baraffe et al. 2015.
Downloaded from BHAC15 site.
Methods
isochrone
([age, metallicity])Extract an individual isochrone from the Baraffe+15 collection.
test_age_interp
(onlineIso, interpIso)Compare one of our interpolated ischrones with one of the isochrones provided online by Baraffe+15.
tracks_to_isochrones
(tracksFile)Create isochrones at desired age sampling (6.0 < logAge < 8.0, steps of 0.01; hardcoded) from the Baraffe+15 tracks downloaded online.
- class evolution.Ekstrom12(rot=True)¶
Bases:
StellarEvolution
Evolution models from Ekstrom et al. 2012.
Downloaded from website.
- Parameters
- rot: boolean, optional
If true, then use rotating Ekstrom models. Default is true.
Methods
create_iso
(ageList[, rot])Given a set of isochrone files downloaded from the server, put in correct iso.fits format for parse_iso code.
format_isochrones
()Parse iso.fits (filename hardcoded) file downloaded from Ekstrom+12 models, create individual isochrone files for the different ages.
isochrone
([age, metallicity])Extract an individual isochrone from the Ekstrom+12 Geneva collection.
- class evolution.Parsec¶
Bases:
StellarEvolution
Evolution models from Bressan et al. 2012, version 1.2s.
Downloaded from here <http://stev.oapd.inaf.it/cgi-bin/cmd>_
Notes
Evolution model parameters used in download:
n_Reimers parameter (mass loss on RGB) = 0.2
photometric system: HST/WFC3 IR channel
bolometric corrections OBC from Girardi+08, based on ATLAS9 ODFNEW models
Carbon star bolometric corrections from Aringer+09
no dust
no extinction
Chabrier+01 mass function
Methods
format_isochrones
(metallicity_list)Parse isochrone file downloaded from Parsec version 1.2 for different metallicities, create individual isochrone files for the different ages.
isochrone
([age, metallicity])Extract an individual isochrone from the Parsec version 1.2s collection.
- class evolution.Pisa¶
Bases:
StellarEvolution
Evolution models from Tognelli et al. 2011.
Downloaded online
Notes
Parameters used in download:
Y = middle value of 3 provided (changes for different metallicities)
mixing length = 1.68
Deuterium fraction: 2*10^-5 for Z = 0.015, 0.03; 4*10^-4 for 0.005
Methods
format_isochrones
(metallicity_list)Rename the isochrone files extracted from Pisa (Tognelli+11) to fit naming/directory scheme
isochrone
([age, metallicity])Extract an individual isochrone from the Pisa (Tognelli+11) collection.
make_isochrone_grid
()Create isochrone grid of given metallicity with time sampling = 0.01 in logAge (hardcoded).